Audio
API Reference
Audio Constraints
The Audio API handles audio interfaces including play and record supported formats.
A Legato device can use several audio interfaces. You choose the input and output interfaces to tie together. The Audio stream related to a particular interface is represented with an 'Audio Stream Reference'.
You can create your own audio path by connecting several audio streams together using audio connectors.
An audio path can support more than two audio interfaces. You can have a basic output audio path of a voice call to connect the Modem Voice Received interface with the Speaker interface, and at the same time, the Modem Voice Received interface can be also connected to a Recorder Device interface.
IPC interfaces binding
All the functions of this API are provided by the audioService.
- Note
- The functions that are highly dependent on the initialization of the lower layers of the audioService will return LE_UNAVAILABLE error code if those layers failed to initialize. Recovery can be attempted by restarting the service.
Here's a code sample binding to audio services:
bindings: { clientExe.clientComponent.le_audio -> audioService.le_audio }
Configure the Audio
The audio profile can be set with the le_audio_SetProfile() function.
- Warning
- Ensure to check the number of supported audio profiles for your specific platform.
An audio profile can be retrieved with le_audio_GetProfile() and set with le_audio_SetProfile().
Then, the following functions let you enable or disable the audio settings on the selected audio interface:
- le_audio_EnableNoiseSuppressor()/le_audio_DisableNoiseSuppressor(): Noise Suppressor.
- le_audio_EnableEchoCanceller()/ le_audio_DisableEchoCanceller(): Echo Canceller.
- le_audio_EnableFirFilter()/ le_audio_DisableFirFilter(): downlink FIR Filter (Finite Impulse Response).
- le_audio_EnableIirFilter()/ le_audio_DisableIirFilter(): downlink IIR Filter (Infinite Impulse Response).
- le_audio_EnableAutomaticGainControl()/ le_audio_DisableAutomaticGainControl(): automatic gain on the selected audio stream.
- le_audio_IsNoiseSuppressorEnabled(): To get the status of Noise Suppressor.
- le_audio_IsEchoCancellerEnabled(): To get the status of Echo Canceller.
To configure the encoding format, use le_audio_GetEncodingFormat() and le_audio_SetEncodingFormat().
To configure gain settings, use le_audio_GetGain() and le_audio_SetGain().
PCM has the following configuration get/set functions:
- le_audio_GetPcmSamplingRate() and le_audio_SetPcmSamplingRate() for Hz rate.
- le_audio_GetPcmSamplingResolution() and le_audio_SetPcmSamplingResolution() for bit/sample settings.
- le_audio_GetPcmCompanding() and le_audio_SetPcmCompanding() for signal settings.
- le_audio_GetSamplePcmChannelNumber() and le_audio_SetSamplePcmChannelNumber() for channel numbers.
To Mute/Unmute the Call Waiting Tone to alert for an incoming voice call when a voice call is already in progress, use le_audio_MuteCallWaitingTone() and le_audio_UnmuteCallWaitingTone().
- Note
- The Call Waiting Supplementary Service must be enabled.
Open/Close an Audio Interface
The following functions let you select the desired interface attributes. Please check the platform constraints in Audio page to ensure that the function is supported before calling it:
- le_audio_OpenMic(): returns an Audio Stream Reference of the analog audio signal coming from the microphone input.
- le_audio_OpenSpeaker(): returns an Audio Stream Reference of the analog audio signal routed to the Speaker output.
- le_audio_OpenUsbRx(): returns an Audio Stream Reference of the digitized audio signal coming from an external device connected via USB Audio Class.
- le_audio_OpenUsbTx(): returns an Audio Stream Reference of the digitized audio signal routed to an external device connected via USB Audio Class.
- le_audio_OpenPcmRx(): it returns an Audio Stream Reference of the digitized audio signal coming from an external device connected via the PCM interface.
- le_audio_OpenPcmTx(): it returns an Audio Stream Reference of the digitized audio signal routed to an external device connected via the PCM interface.
- le_audio_OpenI2sRx(): it returns an Audio Stream Reference of the digitized audio signal coming from an external device connected via the I2S interface.
- le_audio_OpenI2sTx(): it returns an Audio Stream Reference of the digitized audio signal routed to an external device connected via the I2S interface.
- le_audio_OpenModemVoiceRx(): returns an Audio Stream Reference of the digitized audio signal coming from a voice call. The audio format is negotiated with the network when the call is established.
- le_audio_OpenModemVoiceTx(): returns an Audio Stream Reference of the digitized audio signal routed to a voice call. The audio format is negotiated with the network when the call is established.
Multiple users can own the same stream at the same time.
le_audio_GetDefaultPcmTimeSlot() can be called to get the default PCM time slot used on the current platform.
le_audio_GetDefaultI2sMode() can be called to get the default I2s channel mode slot used on the current platform.
Call le_audio_Close() to release it. If several users own the same, corresponding stream reference, the interface will close only after the last user releases the audio stream.
You can configure the PCM interface with the le_audio_SetPcmSamplingRate(), le_audio_SetPcmSamplingResolution() and le_audio_SetPcmCompanding() functions. This function must be called before activating an audio path with the PCM interface, in other words you must call this function before connecting the PCM Stream to a connector.
In addition, the le_audio_GetPcmSamplingRate(), le_audio_GetPcmSamplingResolution() and le_audio_GetPcmCompanding() functions allows you to retrieve the PCM interface configuration.
- Note
- Opening a Legato audio stream doesn’t necessarily interact with the audio HW interface. On most platforms, it only allocates a context in memory. The audio path becomes active when the streams are plugged into a connector.
Control an Audio Stream
Once the users get an Audio Stream reference, they can control it with the following functions:
- le_audio_SetGain(): adjust the gain of an audio stream (gain value is specific to the platform).
- le_audio_GetGain(): retrieve the gain of an audio stream (gain value is specific to the platform).
- le_audio_Mute(): mute an audio stream.
- le_audio_Unmute(): unmute an audio stream.
- Note
- Multimedia (playback and recording) must be controlled separately from the main audio path (Microphone/Speaker, I2S, PCM, USB). Muting/Unmuting a multimedia is done by muting/unmuting the multimedia stream, and not the other connected stream. For example, in case of playback + voice on the speaker, if the user wants to mute all the audio coming out of the speaker, it must mute both the Speaker stream and the playback stream.
- Warning
- Ensure to check the list of supported audio streams for these functions on your specific platform.
In the case your platform can support other gains in your audio subsystem, you can set or get the value of them with the following functions:
- le_audio_SetPlatformSpecificGain(): adjust the value of a platform specific gain in the audio subsystem.
- le_audio_GetPlatformSpecificGain(): retrieve the value of a platform specific gain in the audio subsystem.
- Warning
- Ensure to check the names of supported gains for your specific platform.
Create Audio connectors
You can create your own audio path by connecting several audio streams together.
le_audio_CreateConnector() function creates a reference to an audio connector.
You can tie an audio stream to a connector by calling the le_audio_Connect() function.
You can remove an audio stream from a connector by calling the le_audio_Disconnect() function.
When finished with it, delete it using the le_audio_DeleteConnector() function.
The following image shows how to connect a Player stream to play a file towards a remote end during a voice call.
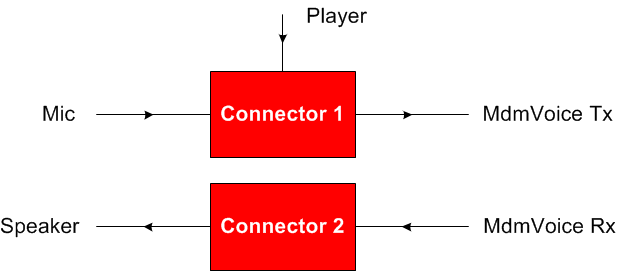
A sample code that implements audio connectors and audio streams during voice call can be found in audioMccTest.c file (please refer to Sample code of audio settings for a dialing call page).
- Note
- It is recommended to set the connectors before the connected streams are used. In particular, a connector using Modem Voice Rx or Tx interfaces has to be created before dialing or answering the call if the application needs to customize the audio path.
Playback
An audio file can be played to any active output interfaces.
Open a player interface by calling:
- le_audio_OpenPlayer(): returns an Audio Stream Reference for file playback. An audio file can be played on the local audio interface like Speaker, USB Tx, PCM Tx, I2S Tx or on the remote audio interface Modem Voice Tx depending the kind of connector (input or output) is tied to.
- le_audio_PlayFile(): plays a specified file. WAVE (Waveform audio file) and AMR (Adaptive Multi Rate) are supported. AMR is an audio compression format optimized for speech coding. Two codecs are supported:
- AMR_NB (AMR Narrowband) codec that encodes narrowband (200--3400 Hz) signals at variable bit rates ranging from 4.75 to 12.2 kbit/s. It was adopted as the standard speech codec by 3GPP.
- AMR-WB (AMR Wideband) is an ITU-T standard speech codec which improved speech quality due to a wider speech bandwidth of 50--7000 Hz.
- le_audio_PlaySamples(): initiates a playback using an audio flow. A pipe has to be opened first, then the PCM samples are sent through the opened pipe. A play can be done only on a connected stream. For instance, the "I2S Tx", "Modem Voice Rx" and "Player" must be previously connected before playing a file. If there are no more PCM samples to be played, the playback must be stopped by calling le_audio_Stop().
Record
- Audio file recording can be done from any active input interface.
Open a "File Recording" interface by calling:
- le_audio_OpenRecorder(): returns an Audio Stream Reference for file recording. The local audio interface like Microphone, USB Rx, PCM Rx, I2S Rx is recorded into an audio file; or the Modem Voice Rx remote audio interface is recorded into an audio file, depending the kind of connector (input or output) is tied to.
- le_audio_RecordFile(): records in a specified file.
- le_audio_SetEncodingFormat(): sets the encoding format. The same formats as the player are supported.
- le_audio_GetEncodingFormat(): gets the encoding format.
- le_audio_GetSamples(): records the audio PCM samples. A pipe has to be opened first, then the PCM samples are sent through the opened pipe. Recording can only be done on a connected stream. For example, the "I2S Rx", "Modem Voice Tx" and "Recorder" must be previously connected before recording a file. If there are no more PCM samples to be retrieved, the recording must be stopped by calling le_audio_Stop().
A PCM configuration must be set with:
- le_audio_SetSamplePcmChannelNumber(): sets the channel number of a PCM sample.
- le_audio_SetSamplePcmSamplingRate(): sets the sampling rate of a PCM sample.
- le_audio_SetSamplePcmSamplingResolution(): sets the sampling resolution (in bits per sample) of a PCM sample.
The PCM samples configuration can be retrieved with:
- le_audio_GetSamplePcmChannelNumber(): gets the channel number of a PCM sample.
- le_audio_GetSamplePcmSamplingRate(): gets the sampling rate of a PCM sample.
- le_audio_GetSamplePcmSamplingResolution(): can be called to get the sampling resolution (in bits per sample) of a PCM sample. The default configuration is PCM 16-bit audio @ 8KHz one channel.
An AMR configuration must be set with:
- le_audio_SetSampleAmrMode(): sets the AMR mode (NB/WB, bitrate).
- le_audio_SetSampleAmrDtx(): can be called to activate/deactivate the Discontinuous Transmission (DTX) to reduce bandwidth usage during silence periods. The AMR configuration can be retrieved with:
- le_audio_GetSampleAmrMode(): gets the AMR mode.
- le_audio_GetSampleAmrDtx(): gets the Discontinuous Transmission (DTX).
To stop a play/record call le_audio_Stop():
- The playback/record stops playing/recording, and the read/write position indicator associated with the file stream is rewound to the beginning of the file. A new file can be played/recorded using le_audio_PlayFile()/le_audio_PlaySamples()/le_audio_RecordFile()/le_audio_GetSamples().
- le_audio_Pause(): can be called to pause a play/record. The file playing/recording is put on hold, the read/write position indicator of the file is not moved.
- le_audio_Resume(): can be called to resume a paused play/record. The file playing/recording continues at the file's position indicator held after the pause.
- le_audio_Flush(): can be called to flush the remaining audio samples before sending them to the audio driver.
You can also register a handler function for media-related notifications like errors or audio events.
le_audio_AddMediaHandler() function installs a handler for player/recorder stream notifications.
le_audio_RemoveMediaHandler() function removes the player/recorder handler function.
- Note
- The
LE_AUDIO_MEDIA_NO_MORE_SAMPLES
event indicates when all samples put into the pipe by the user's App have been sent to the audio driver (see le_audio_PlaySamples()).
A sample code that implements audio playback and capture can be found in audioPlaybackRec.c file (please refer to Sample code of audio playback and capture page).
DTMF
The le_audio_PlayDtmf() function allows the application to play one or several DTMF on a playback stream. The duration and the pause of the DTMFs must also be specified with the input parameters.
The le_audio_PlaySignallingDtmf() function allows the application to ask the Mobile Network to generate on the remote audio party the DTMFs. Compared with le_audio_PlayDtmf(), le_audio_PlaySignallingDtmf() function may offer a better signal quality, but the the duration and the pause timings may be less accurate.
The application must register a handler function to detect incoming DTMF characters on a specific input audio stream. The le_audio_AddDtmfDetectorHandler() function installs a handler for DTMF detection.
The le_audio_RemoveDtmfDetectorHandler() function uninstalls the handler function.
The DTMFs are: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, *, #, A, B, C, D. Not case sensitive.
- Note
- The DTMF decoding works only on an active audio path.
A sample code that implements DTMF playback and decoding can be found in audioDtmfTest.c file (please refer to Sample code of dtmf playback and decoding page).
Copyright (C) Sierra Wireless Inc.