Software Update
API Reference
Update Process
Update Pack Format
Use Software Update
This API uses Update Pack Format to update a target device software/firmware.
Update packs can contain one or more tasks to be performed by the Update Daemon.
From the client view, the update service follows this state machine while doing an update:
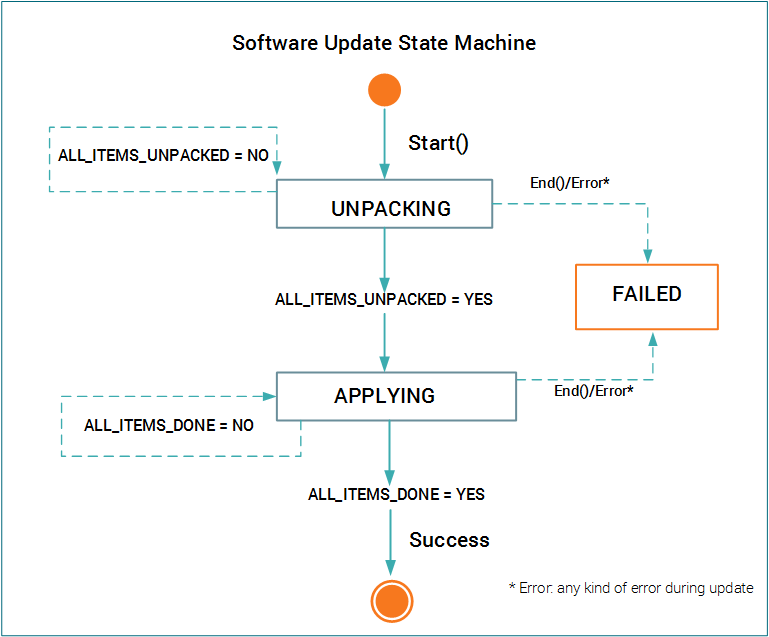
API Usage Guidelines
Typically, the API is used like this:
- Client calls le_update_Start() providing a file descriptor for the update service to read the update pack and a notification callback function to call with updates.
- Progress reports are sent to the client periodically through the notification function.
- If the update fails, le_update_GetErrorCode() can be used to find out more info.
- When the client is finished with the update, the client MUST call le_update_End() to deallocate resources.
To cancel an update before it finishes, call le_update_End().
If the client disconnects before ending the update session, the session will automatically end. If the update is still in progress, it may be cancelled (if it's not too late).
Sample Code
This C code sample calls an update service provider API to perform an update:
void SoftwareUpdate(void){int fd = 0; // Update data coming via STDINle_result_t result = le_update_Start(fd, UpdateProgressHandler, NULL);if (result != LE_OK){fprintf(stderr, "Update refused by server. Try again later.\n");// NOTE: It's okay to not delete the update here because we are exiting, so the handle// will be deleted automatically.exit(EXIT_FAILURE);}}// Sample callback function implementation.static void UpdateProgressHandler(le_update_State_t updateState, ///< Current state of the update.uint32 percentDone, ///< Percent done for current state.void* contextPtr ///< Context pointer (NULL).){switch(updateState){fprintf(stdout, "Unpacking: %d%% \n", percentDone);break;break;case LE_UPDATE_STATE_APPLYING:fprintf(stdout, "Applying: %d%% \n", percentDone);break;case LE_UPDATE_STATE_SUCCESS:fprintf(stdout, "\nSUCCESS\n");exit(EXIT_SUCCESS);case LE_UPDATE_STATE_FAILED:fprintf(stderr, "\nFAILED: error code %d\n", le_update_GetErrorCode());exit(EXIT_FAILURE);}}
Installed System Information
It is possible to get the index and hash for all of the currently installed systems. The following is an example of how one would list all installed systems and their hashes.
int32_t systemIndex = le_update_GetCurrentSysIndex();do{char hashBuffer[LE_LIMIT_MD5_STR_LEN + 1];{LE_INFO("System: %d -- %s", systemIndex, hashBuffer);}else{LE_ERROR("System: %d -- NOT FOUND", systemIndex);}}while ((systemIndex = le_update_GetPreviousSystemIndex(systemIndex)) != -1);
Copyright (C) Sierra Wireless Inc.